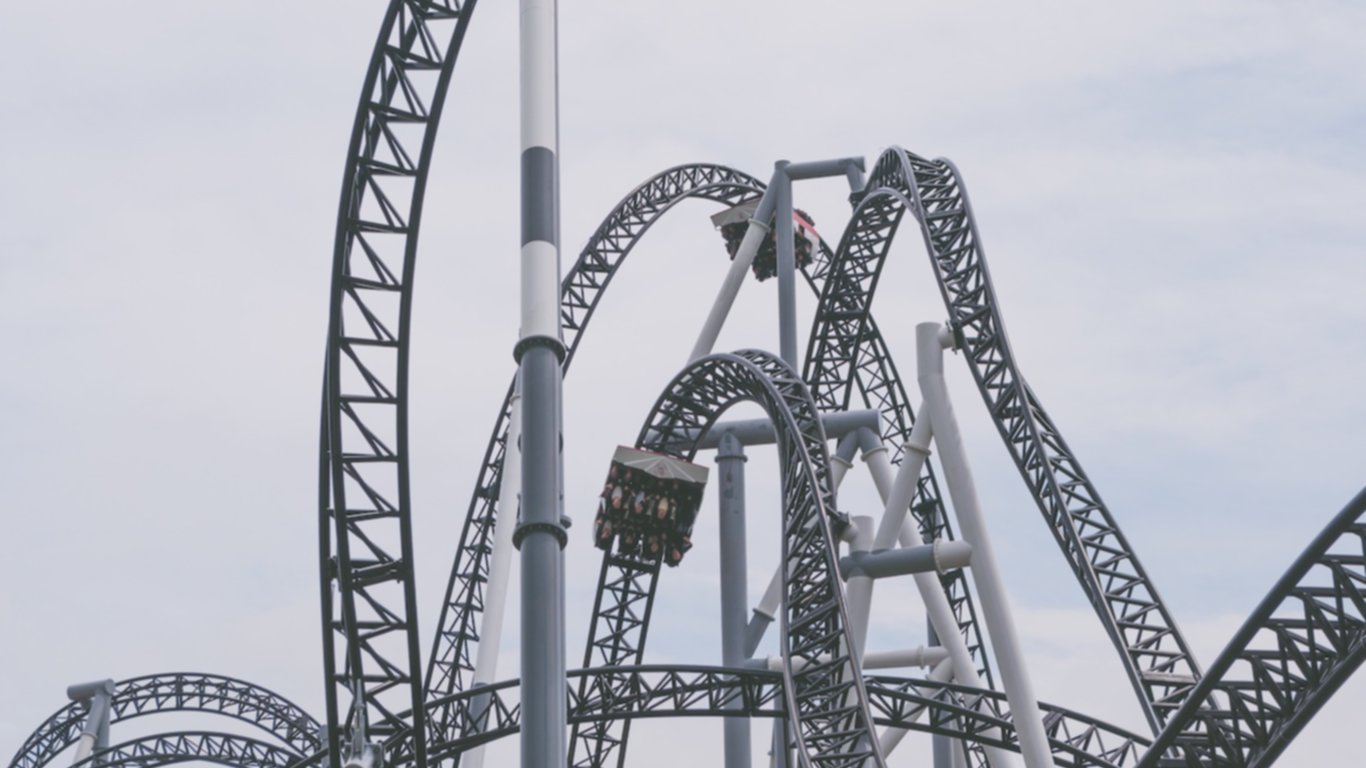
Rails 5 was finally released, so it’s time to do an overview of all the awesome new stuff we’ve got to play with. The first thing I want to talk about is the brand new Rails 5 API mode - it lets us build web APIs without all the clutter usually included in the controllers.
What’s a Web API?
APIs are Application Programming Interfaces – basically, an interface for two softwares to communicate. Web APIs are the same thing, but are usually accessible in the World Wide Web through HTTP or similar protocols.
Web APIs are often added to web applications to offer a programmatic way to use features or retrieve data. These days, it’s also quite common to build web APIs that will only be used as backends for mobile or JavaScript applications.
Web APIs usually offer JSON (or XML) representations instead of HTML. JSON documents are easier to understand and use for an automated client, like the ones mentioned above.For example, Reddit offers a public API.
A Short History
This new feature doesn’t come out of nowhere. It’s actually the Rails-API project that was merged into Rails 5.
The discussion thread on GitHub, initiated by DHH but encouraged by Santiago Pastornio, is interesting to follow and available here.
How Does It Work?
Creating a Rails API-only application is super simple. The command is:
rails new my_app --api
This will create a stripped-down Rails application with a smaller set of middleware. It will also configure the generators to skip the views/helpers/assets and change the parent for ApplicationController
from ActionController::Base
to ActionController::API
.
The following line is added to the config/application.rb
file, and sets the application as a web API only:
# config/application.rb
module MyApp
class Application < Rails::Application
config.api_only = true
end
end
After that, the development is pretty similar to what you’re used to with Ruby on Rails. You won’t have views, helpers, or assets because all of these will be off-loaded to the clients. To replace views, you will probably want to use some kind of serializers to build the JSON documents.
Why Use Rails API Mode Instead of Sinatra or Grape?
People often wonder why use Rails to build a web API, rather than something smaller and simpler like Grape or Sinatra. While those frameworks are fine for small applications, you will quickly find the need to add libraries in order to add some mandatory features like an ORM, some representation builders, an auto-reload feature, etc. In the end, you will have something as big as a Rails application, just made up of various libraries.
That’s not necessarily a bad thing. With this approach, you can pick exactly which gems you want to use. But deciding to go with Rails is also a viable alternative, since you get all those features from the get go.
Plus, Rails handles a lot of stuff that you may be used to, and that you won’t find in smaller frameworks (security, conditional GETs, caching, etc.).
When Should You Use It?
You should only use Rails 5 API mode if your application is a web API. This means you won’t be sending HTML representations, but instead only JSON (or XML) which will be used by automated clients.
Differences From a Traditional Rails Application
Outside of the lack of views, helpers and assets, the two main differences between Rails APIs and regular Rails applications are the middleware used and the module included in the controllers.
Middleware
Rack middleware are an implementation of the pipeline design pattern, and are heavily used in Ruby on Rails to perform actions on the request and response objects.
By default, a Rails API-only application comes with a limited set of middleware. This is meant to increase the throughput by removing unnecessary functionalities.
Adding a middleware is as simple as adding a new line in the application.rb
file. For example, if you’d like to add Rack::Deflater to your API:
# config/application.rb
module Alexandria
class Application < Rails::Application
config.api_only = true
config.middleware.use Rack::Deflater
end
end
To remove one of the default middleware, use delete
instead of use
. Let’s remove ActionDispatch::Static, used to serve static files from the public folder.
# config/application.rb
module Alexandria
class Application < Rails::Application
config.api_only = true
config.middleware.delete ActionDispatch::Request
end
end
You can see the list of middleware used in your Rails application with the following command:
rails middleware
You can learn more about the middleware included in your application here.
Controllers
The ActionController::API
, from which the ApplicationController
inherits in an API-only application, also comes with fewer modules than the regular ActionController::Base
.
This allows requests to be processed faster and reduce the response time of your web APIs.
Going further
If you want to dig deeper into Rails 5 API mode, take a look at Master Ruby Web APIs. In this book, you will learn the basics of building web APIs with Sinatra before going on to build a complete e-commerce API with Rails 5 API mode.