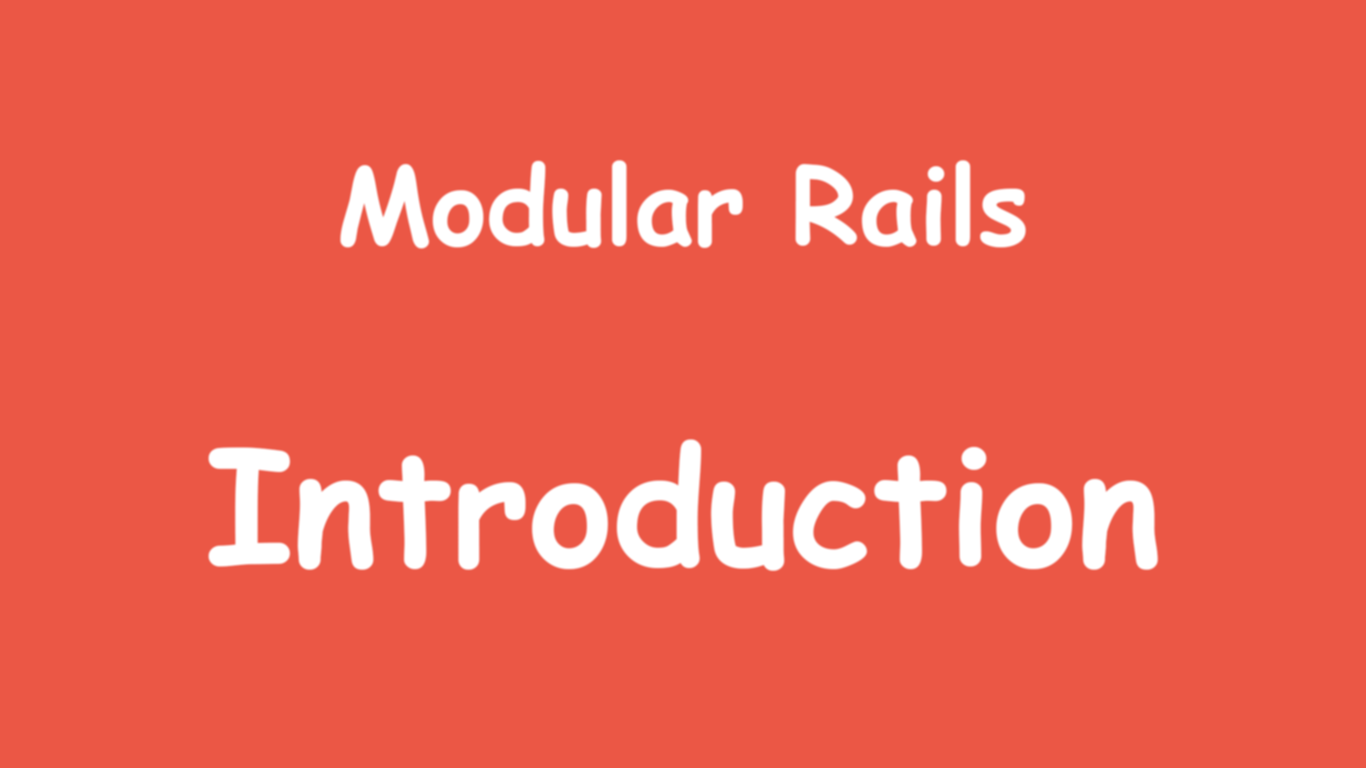
When you first create a Rails app, you can just follow the conventions provided by Ruby on Rails and your development will be smooth and nice. But after months, or years, your app can become cumbersome.
And now it’s :
- Hard to maintain.
- Hard to improve.
- Hard to test.
Your app has just become a pain in the a.**
From this point, you’ve got a few choices.
You could just give up, but I’m sure you’re braver than that. You could also divide your web application in a set of different applications and APIs, but that sound like a lot of work. Or maybe you can rewrite your app in a more modular way.
Note that if you’re just starting to build your application/framework/library, you might want to start clean by building it with modularity in mind. Keep reading. ;)
First, what’s modularity ?
Here’s what Wikipedia has to say about it :
Modular programming is a software design technique that emphasises separating the functionality of a program into independent, interchangeable modules, such that each contains everything necessary to execute only one aspect of the desired functionality. Conceptually, modules represent a separation of concerns, and improve maintainability by enforcing logical boundaries between components.
Basically, it’s all about isolating the functionalities/features of your application into independent and interchangeable components.
Let me give you an example of a real life Ruby on Rails application. We’re going to use a CMS, like Wordpress, as an example.
In a regular monolithic application (as opposed to modular application), we’d probably have the following files (with a very simplified architecture) :
app/
assets/
controllers/
application_controller.rb
sessions_controller.rb
users_controller.rb
posts_controller.rb
comments_controller.rb
models/
user.rb
post.rb
comment.rb
helpers/
...
views/
posts/
...
comments/
...
admin/
posts/
comments/
You get the idea. Just a few controllers and models to handle users, posts and comments. Now, we can already identify 3 different functionalities : the user system, the posts and the comments.
So how do we divide those features in different modules with Ruby on Rails ?
We use Ruby on Rails engines ! By using Rails engines the way I’m going to show you, the actual app is nothing more than an empty shell. All the content is actually inside the engines :
your_app/
app/
... # mostly empty
config/
...
engines/
core/
app/
controllers/
application_controller.rb
sessions_controller.rb
users_controller.rb
models/
user.rb
views/
users/
post/
app/
controllers/
posts_controller.rb
models/
post.rb
views/
posts/
comment/
app/
controllers/
comments_controller.rb
models/
comment.rb
views/
comments/
As you can see, we put the user system inside the Core engine. The Core, as the name implies, contains the minimum logic for our app to run. We can then add features on top of it by extending its controllers, models and views inside the Post and Comment engines. The engines are loaded through the Gemfile of the main Rails application.
Want to remove the comments from your blog ? You can just remove this engine from the Gemfile. Your app will still be working perfectly fine since the Comment engine was completely encapsulated. The main app and the other engines will still be working correctly.
That’s the basic idea behind modularity.
Advantages
Using Rails engines in this way come with a set of advantages. Here are a few of those :
- You can easily add or remove feature since those are completely isolated.
- Reusability. The core that I show you before can easily be reused in any application. Same can be said for some feature engines.
- Each engine includes its own tests, so each feature is tested separately.
Want to know more ?
You can read about the use cases for modularity here.
You can also checkout the book I wrote about modular applications : Modular Rails.
Don’t hesitate to subscribe to my newsletter in the form below or on the book website to receive a free sample of the book.
Here are the other posts of the series: